diff --git a/scripts/README.md b/scripts/README.md
index d869df5..054d86e 100644
--- a/scripts/README.md
+++ b/scripts/README.md
@@ -26,3 +26,7 @@ Description
- tictactoe
A cli-based tictactoe game to play with the computer.
[Rounak Vyas](http://www.github.com/itsron717)
+
+- eyetracking
+ An advanced eye-tracking system with OpenCV and data extracted from a recorded .flv file. The program bounds the box of the iris and scales on the axis.
+ [Akash Ramjyothi](https://github.com/Akash-Ramjyothi)
diff --git a/scripts/eyetracking/README.md b/scripts/eyetracking/README.md
new file mode 100644
index 0000000..a8e75a1
--- /dev/null
+++ b/scripts/eyetracking/README.md
@@ -0,0 +1,10 @@
+# Advanced-Eye-Tracking
+
+Developed an advanced eye-tracking system with OpenCV and data extracted from a recorded .flv file. The program bounds the box of the iris and scales on the axis.
+
+## Sample Demo:
+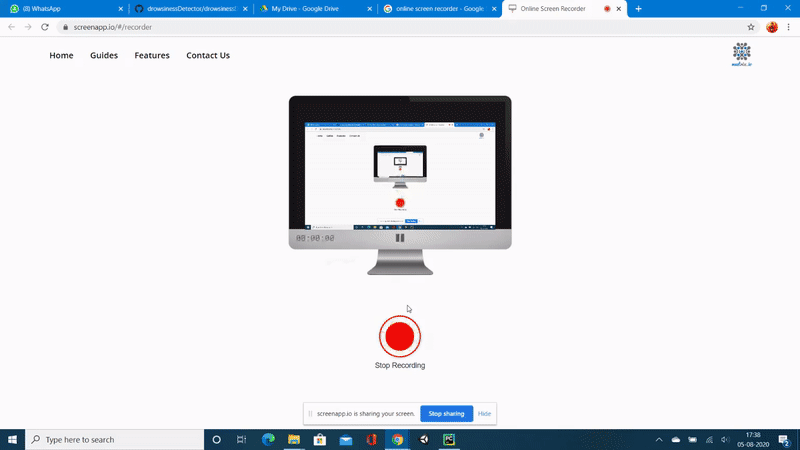
+
+## Usage
+`python main.py`
+
diff --git a/scripts/eyetracking/eye_recording.flv b/scripts/eyetracking/eye_recording.flv
new file mode 100644
index 0000000..2d9afa2
Binary files /dev/null and b/scripts/eyetracking/eye_recording.flv differ
diff --git a/scripts/eyetracking/main.py b/scripts/eyetracking/main.py
new file mode 100644
index 0000000..4463376
--- /dev/null
+++ b/scripts/eyetracking/main.py
@@ -0,0 +1,36 @@
+import cv2
+import numpy as np
+
+cap = cv2.VideoCapture("scripts/eyetracking/eye_recording.flv")
+
+while True:
+ ret, frame = cap.read()
+ if ret is False:
+ break
+
+ roi = frame[269: 795, 537: 1416]
+ rows, cols, _ = roi.shape
+ gray_roi = cv2.cvtColor(roi, cv2.COLOR_BGR2GRAY)
+ gray_roi = cv2.GaussianBlur(gray_roi, (7, 7), 0)
+
+ _, threshold = cv2.threshold(gray_roi, 3, 255, cv2.THRESH_BINARY_INV)
+ _, contours, _ = cv2.findContours(threshold, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
+ contours = sorted(contours, key=lambda x: cv2.contourArea(x), reverse=True)
+
+ for cnt in contours:
+ (x, y, w, h) = cv2.boundingRect(cnt)
+
+ #cv2.drawContours(roi, [cnt], -1, (0, 0, 255), 3)
+ cv2.rectangle(roi, (x, y), (x + w, y + h), (255, 0, 0), 2)
+ cv2.line(roi, (x + int(w/2), 0), (x + int(w/2), rows), (0, 255, 0), 2)
+ cv2.line(roi, (0, y + int(h/2)), (cols, y + int(h/2)), (0, 255, 0), 2)
+ break
+
+ cv2.imshow("Threshold", threshold)
+ cv2.imshow("gray roi", gray_roi)
+ cv2.imshow("Roi", roi)
+ key = cv2.waitKey(30)
+ if key == 27:
+ break
+
+cv2.destroyAllWindows()